Using a new feature called Version Tolerant Serialization in .NET 2, it becomes possible to change class definitions and still deal with old serialized data versions after an application has been updated. This is actually extremely easy to do. Consider this code:
[Serializable]
public class Test {
string name;
public string Name {
get { return name; }
set { name = value; }
}
}
Now say you have deployed version 1 of your application with this class definition and us …
This page describes a problem with .NET simple data binding — a property of a custom type couldn’t be bound correctly to the Text
property of a standard TextEdit, because the internal logic would never convert the string value back to the object type. A given TypeConverter
would simply be ignored in the process. The only workaround was to use the Parse
event of the Binding
object — not a nice way to go because the code wouldn’t be central to the class and its type converter any more.
…
Just saw this post in a newsgroup about how to show the horizontal lines that Microsoft likes to use in their dialogs. A lot of people replied with ideas of using other controls, like panels, to achieve the desired effect — to me, a much simpler and more effective idea is to just create a control myself. So, the specific style described by the OP seems to be this:
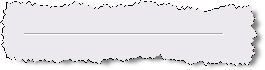
This is what you get by using a label set to a height of 2 and with a _BorderSt …
Just bragging :-) Here it is: The Daily Grind 725
If you haven’t seen my Electric Editing plugin, look here.
Update: I just noticed that Jim Holmes also wrote a report about it on Visual Studio Hacks.
This is the seventh article in my mini series about object pooling. Be sure to read part 1, part 2, part 3, part 4, part 5 and part 6 first. As some of you may have noticed, I introduced a bad bug in the last article about growing and shrinking, specifically in the code that would call the ExtendPoolBy
and ShrinkPoolBy
methods. The amount by which to grow or shrink the pool was calculated as the difference between two percentage figures and passed in to the two methods, which really expecte …
This is the sixth article in my mini series about object pooling. Be sure to read part 1, part 2, part 3, part 4 and part 5 first. It took me a while to find the time for the next article, but here it is. Now we’re finally going to deal with the topic of growing and shrinking the pool. One of the first questions we have to ask when it comes to this is, when are we going to do it? We have one mechanism for growing the pool implemented already, which takes place when, during a call to the `GetObj …
It’s next week and I’m going to be there. Registration is free, so if you’re at all interested in project management or anything that has remotely to do with it, be sure to check out the website and consider coming!
This is the fifth article in my mini series about object pooling. Be sure to read part 1, part 2, part 3 and part 4 first. This part is going to make some modifications to the code in the GetObject
method to implement various alternative behaviours there. Think about it: what do you want to happen if there’s no free object to be had in the pool? I came up with the following possible options:
- Return
null
. In this case, the caller would have to deal with the problem further if it can’t ge …